Git
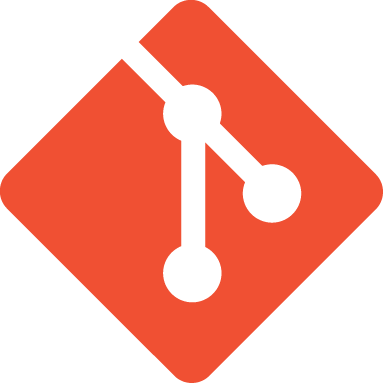
Robert Rouhani
Why?
- History
- Backups
- Collaboration
- Lightweight
How?
GitHub

BitBucket

Command line Git
Create a local repository, add a file, commit it
$ mkdir new_project && cd new_project
$ git init
$ echo "My new project" > README.txt
$ git add .
$ git commit -m"Initial commit."
Breakdown
$ mkdir new_project && cd new_project
Creates a new directory called new_project and makes it the current directory.
Breakdown
$ git init
Initializes a git repository by creating the .git/ folder in the current directory. Unlike SVN, .git/ only exists in the root dir
Breakdown
$ echo "My new project" > README.txt
Writes the string "My new project" to the file README.txt
Normally would be making changes to your code
Breakdown
$ git add .
Git has a "staging area". This allows you to only commit parts of the changes you have made.
"." adds all the changes to the staging area, can be individual files like "README.txt" or even blocks in a file with the "-p" flag.
breakdown
$ git commit -m"Initial commit."
Stores a snapshot of the repository with the added changes. Tracked with a SHA1 hash.
Each commit stores the SHA1 hash of it's "parent" to create a tree of commits.
You can always revert to an older version (unless you put some effort into rewriting your repo's history)
branching
$ git branch newbranch
$ git checkout newbranch
$ echo "a new file" > file.txt
$ git add .
$ git commit -m"Committing on a new branch"
$ git checkout master
$ git merge newbranch
Breakdown
$ git branch newbranch
Creates a new branch (very cheap to do!)
Following our old example, these are our branches:
$ git branch
* master
newbranch
breakdown
$ git checkout newbranch
Switch to the new branch, so that we can make changes on it.
Current branches:
$ git branch
master
* newbranch
Breakdown
$ echo "a new file" > file.txt
$ git add .
$ git commit -m"Committing on a new branch"
Same as before, make changes, add them, commit it.
Branches are pointers to commits, master stays at "Initial commit." but newbranch points to the SHA1 checksum for "Committing on a new branch"
breakdown
$ git checkout master
$ git merge newbranch
Checkout to master again (now your working directory is back at "Initial commit.")
Then merge the two sets of changes together. In this example, the changes are completely independent, so master "fast-forwards" to the commit that newbranch is pointing to.
Remotes
What if you have collaborators?
$ git remote add origin https://github.com/user/repo.git
$ git push -u origin master
Specifies a URL as one that has the same repository.
Then it sends the local copy of the history to the remote.
Remotes
$ git fetch origin
$ git remote add my-desktop git@mydesktop:repo.git
$ git fetch my-desktop
You can fetch changes (downloads commits with SHA1 hashes that you don't have on your machine) from multiple places.
(De)Centralization
Git does not rely on a central server to operate.
It is possible to push/pull code directly to/from collaborators.
It is usually beneficial to also have a central copy, though.
GitHub and BitBucket provide that.
GitHub
Provides a copy of your repo accessible from anywhere
Lets you easily clone a repository, make changes, and then ask the maintainer to merge them in
Gives you all sorts of extra features like free web hosting for static sites, a wiki for your project, etc.
github - forking

Press the "Fork" button on any repo, it will create a copy under your account:

Github - Pull Request
After you fix something, ask the author to fetch the changes from your copy and merge them in to theirs.

Popular Git clients
If you're not a huge fan of the command line, there are several clients out that provide a nice interface to everything.
- GitHub for Windows/Mac
- SourceTree
- TortoiseGit
- Tower
- Extension/Plugin for your favorite IDE!
GitHub for Windows
GitHub for Mac

SourceTree

TortoiseGit

Tower

IDE Plugins/extensions
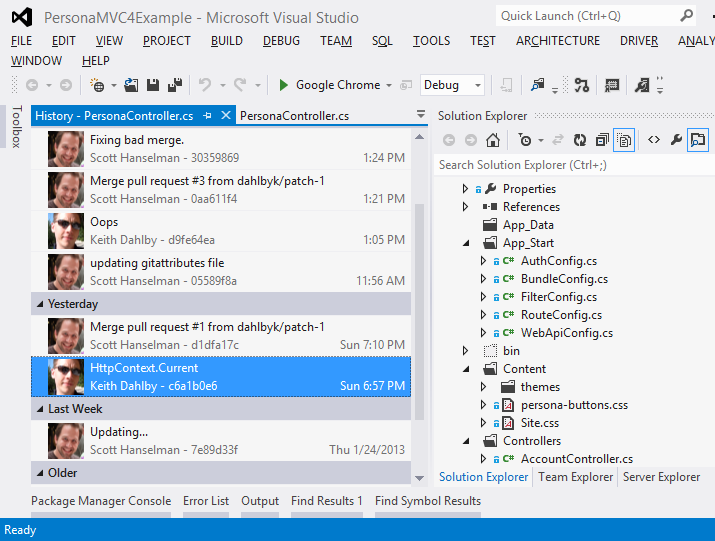
Git Flow

git flow
- master is always production-ready
- hotfixes only contain severe bug fixes
- release branches are for bugfixes leading to release on master
- develop contains finished but untested features and bugfixes
- feature branches are the main hacking branches, only one feature per branch
git flow

git flow
I recommend reading the original blog post:
Thanks
Professor Goldschmidt
Professor Moorthy
Sean O'Sullivan
RCOS
QUestions?
Git
By Robert Rouhani
Git
- 2,551