Styled Components
Why and How

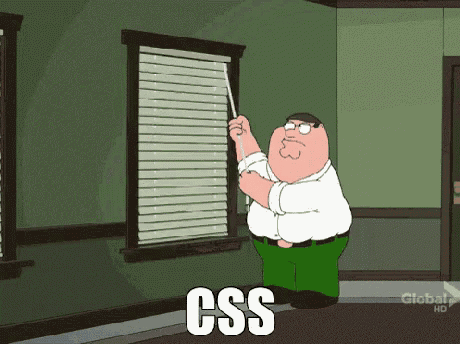
Stylesheets are like a gas.
- They like to expand
- They can cause explosions
- They tend to stink
The utopia
- Repeatable / composable
- Easy refactoring
- Programming concepts (functions, variables etc)
So where's my utopia?
Embracing the Tight Coupling
- A UI component is
Structure + Styles + Interativity - HTML + CSS + JS
- JSX acknowledges this for HTML/CSS
- Doesn't really solve the CSS issue
out of the box
const MyComponent = ({children}) => (
<div style="border: 1px dotted dodgerblue; background-color: whitesmoke;">
{children}
</div>
)
//... usage
return (
<div>
<MyComponent>
This is a styled component
</MyComponent>
<MyComponent>
This is another styled component
</MyComponent>
</div>
)
<div>
<div style="border: 1px dotted dodgerblue; background-color: whitesmoke;">
This is a styled component
</div>
<div style="border: 1px dotted dodgerblue; background-color: whitesmoke;">
This is another styled component
</div>
</div>
const fancyStyles = {
border: '1px dotted dodgerblue',
backgroundColor: 'whitesmoke'
}
const MyFancierComponent = ({children}) => (
<div style={fancyStyles}>
{children}
</div>
)
//... usage
return (
<div>
<MyComponent>
This is a styled component
</MyComponent>
<MyComponent>
This is another styled component
</MyComponent>
</div>
)
<div>
<div style="border: 1px dotted dodgerblue; background-color: whitesmoke;">
This is a styled component
</div>
<div style="border: 1px dotted dodgerblue; background-color: whitesmoke;">
This is another styled component
</div>
</div>
Cons
- Output size
- Not composable/extendable
- No pseudo selectors/elements
- Can't read from state/props
With
styled-components
import styled from 'styled-components';
const StyledComponent = styled.div`
border: 1px dotted dodgerblue;
background-color: whitesmoke;
`
//...composable too!
const ExtendedComponent = styled(StyledComponent)`
padding: 35px;
`
//... usage
return (
<div>
<StyledComponent>
This is a styled-components component
</StyledComponent>
<StyledComponent>
This is another styled-components component
</StyledComponent>
<ExtendedComponent>
This is an extended styled-components component
</ExtendedComponent>
</div>
)
<div>
<div class="sc-hMqMXs">
This is a styled-components component
</div>
<div class="sc-hMqMXs">
This is another styled-components component
</div>
<div class="sc-iAyFgw">
This is an extended styled-components component
</div>
</div>
Caveat: `styled(Element)` works like a SASS mixin
Pros
- Optimised stylesheet
- HTML/JS/CSS in context
- Standard CSS syntax (+ nesting + JS!)
- Easy to refactor
Examples
Thanks!
💅
Styled Components
By juanojeda
Styled Components
- 368