Awesome Ruby 1
By:
Sameer Rahmani (@lxsameer)
From:

Who am I ?
I ...
- have about 12 years experience in programming
- have over thousands of contributes to FOSS world
- am official GNU project developer
- am a member of Debian Python team
- am an expert on:
C/C++ , Python, PHP, Perl, Javascript, Clojure, Lisp, Lua

www.iranonrails.ir
Iranian Ruby users group
What Can we do with
Ruby?
Desktop Applications

Mobile Applications
- Ruby Motion (iOS)
- Rhodes
- Apache Flex
- Susanoo
- And many others
Robotics & Electronics

and more ...
Web Apps


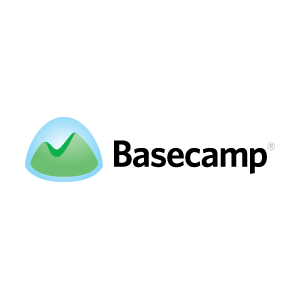
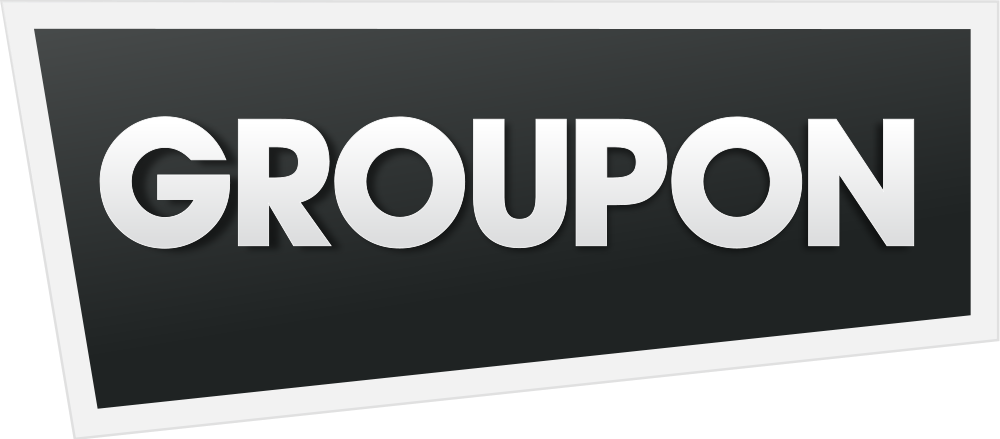
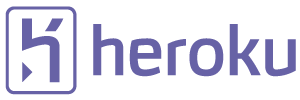


Enything you want
Why Ruby ?

First of all it's FREE
Ruby is designed to make programmers happy.
- Matz
Ruby brings large increase in productivity
With Non traditional programming style
Ruby is easy to
Learn
&
Develop
Too many resources to learn Ruby
Huge community. I mean Huuuge
Lots of useful Gems
Documentation
Ruby knowledge is in demand
Beautiful code
RubyOnRails
And other tools like
- EventMachine
- Artoo
- Sinatra
- Sidekiq
- Rescue
- ....

King of
Meta Programming
Every thing is an object
string = String.new
2.times do
puts "Hello world"
end
# Hello world
# Hello world
1.upto(100) { |i| puts i }
# 1
# 2
...
# 100
some_list = [String, "some string", Integer]
Everything is an Object
class Class
def some_method
puts "I'm in 'Class' class"
end
end
class Test
end
Test.some_method
# I'm in 'Class' class
Blocks
people.each do |person|
if person.sick?
doctor.visit person
else
person.jumps_around
end
end
hello = Proc.new do |string|
puts "Hello #{string.upcase}"
end
hello.call 'sameer'
# Hello sameer
Open Classes
class String
def say_hello
puts "Hello #{self.to_s}"
end
end
"sameer".say_hello
# Hello sameer
That's enough
Getting Start
Installing Ruby
Solutions
- Installing Ruby directly
- Using RVM
- Using `rbenv`
- Using `rbch`
Use ruby installer on MS Windows
Installing using `rbenv`
You need `git` and `build-essentials`
$ git clone https://github.com/sstephenson/rbenv.git ~/.rbenv
$ echo 'export PATH="$HOME/.rbenv/bin:$PATH"' >> ~/.bashrc
$ echo 'eval "$(rbenv init -)"' >> ~/.bashrc
$ git clone https://github.com/sstephenson/ruby-build.git \
~/.rbenv/plugins/ruby-build
Now let's install ruby 2.1.4 and make it default
$ rbenv install 2.1.4
$ rbenv versions
system
2.1.4
$ rbenv global 2.1.4
$ rbenv versions
system
*2.1.4
Basic concepts
- How the ruby program works?
- How ruby runs programs?
- How can I try ruby easily?
- What is `gem`?
- What is `bundler`
The fun part
puts 'Hello World'
Objects
- "Things" in Ruby are Objects
- These "things" come in types called Classes
String, Integer, Class, ActiveRecord::Base
Methods
Methods are verbs - Actions and operations on objects (things)
[7] pry(main)> "IranOnRails".reverse
=> "sliaRnOnarI"
[8] pry(main)> 5.even?
=> false
[9] pry(main)>
Methods
- Sometimes methods take parameters.
- Parentheses around parameter are optional
[11] pry(main)> "IranOnRails rocks".sub('IranOnRails', 'Karajlug')
=> "Karajlug rocks"
[12] pry(main)> "IranOnRails rocks".sub 'IranOnRails', 'Karajlug'
=> "Karajlug rocks"
[13] pry(main)>
Kernel Methods
Some of the basic methods are available everywhere
gets # Read a string from terminal (stdin)
puts "Hey guys"
exit
Variables
- Name for objects (things)
- Place to store things we'll want later
name = "Sameer"
nickname = 'lxsameer'
twitter = "@" + nickname
PI = 3.14
Strings
[14] pry(main)> "Ruby".length
=> 4
[15] pry(main)> "Ruby".upcase
=> "RUBY"
[16] pry(main)> "Ruby".upcase.capitalize
=> "Ruby"
[17] pry(main)> "Ruby" + "onRails"
=> "RubyonRails"
[18] pry(main)> rails = "onRails"
=> "onRails"
[19] pry(main)> "Ruby#{rails}"
=> "RubyonRails"
[20] pry(main)> "Ruby on Rails".split(' ')
=> ["Ruby", "on", "Rails"]
[21] pry(main)>
Arrays
List of objects (things)
[22] pry(main)> langs = ["Ruby", "Python", "Clojure", "Scala", "C"]
=> ["Ruby", "Python", "Clojure", "Scala", "C"]
[23] pry(main)> langs.count
=> 5
[24] pry(main)> langs.sort.join(', ')
=> "C, Clojure, Python, Ruby, Scala"
[25] pry(main)> langs[3]
=> "Scala"
[26] pry(main)> langs[-1]
=> "C"
[27] pry(main)> langs[1..3]
=> ["Python", "Clojure", "Scala"]
[28] pry(main)>
Hashes
Key/Value associations
[28] pry(main)> frameworks = { "Ruby" => "RubyOnRails",
"Python" => "Django" }
=> {"Ruby"=>"RubyOnRails", "Python"=>"Django"}
[29] pry(main)> frameworks["Ruby"]
=> "RubyOnRails"
[30] pry(main)> frameworks["PHP"]
=> nil
[31] pry(main)> frameworks
=> {"Ruby"=>"RubyOnRails", "Python"=>"Django"}
[32] pry(main)>
Conditionals
def analyze(page)
fail '"page" is nil' if page.nil?
if page.even?
puts 'Page is even'
else
puts 'Page is odd'
end
end
analyze(10)
- Everything in ruby has a "truth value"
- Things that are considered false: false, nil
Loops
5.times { puts "Hey" }
1.upto(10).each { |i| puts i }
i = 0
while i <= 10
puts i
i += 1
end
for i in (1..10)
puts i
end
(1..10).each do |i|
puts i
end
["Ruby", "Python", "Lisp"].each do |i|
puts i
end
Symbols !
[57] pry(main)> "Ruby".object_id
=> 70043392889260
[58] pry(main)> "Ruby".object_id
=> 70043393002600
[59] pry(main)> "Ruby".object_id
=> 70043393124120
[60] pry(main)> "Ruby".object_id
=> 70043393245640
[61] pry(main)> "Ruby".object_id
=> 70043393399880
[62] pry(main)> :Ruby.object_id
=> 3904908
[63] pry(main)> :Ruby.object_id
=> 3904908
[64] pry(main)> :Ruby.object_id
=> 3904908
[65] pry(main)> :Ruby.object_id
=> 3904908
[66] pry(main)>
That's it for today
Whaaat ? I want to learn more
- tryruby.org
- Online schools (CodeAcademy, CodeSchool)
- Local courses
- Also docs and books are always out there
Get help
IranOnRails community ML
IRC channel on freenode:
- #iranonrails
- #ruby
- #rubyonrails
Where to find me
Website:
Email:
lxsameer => gnu.org
Twitter:
@lxsameer
I'm always available on IRC:
#5hit #iranonrails
The End
Awesome Ruby 1
By Sameer Rahmani
Awesome Ruby 1
- 2,139